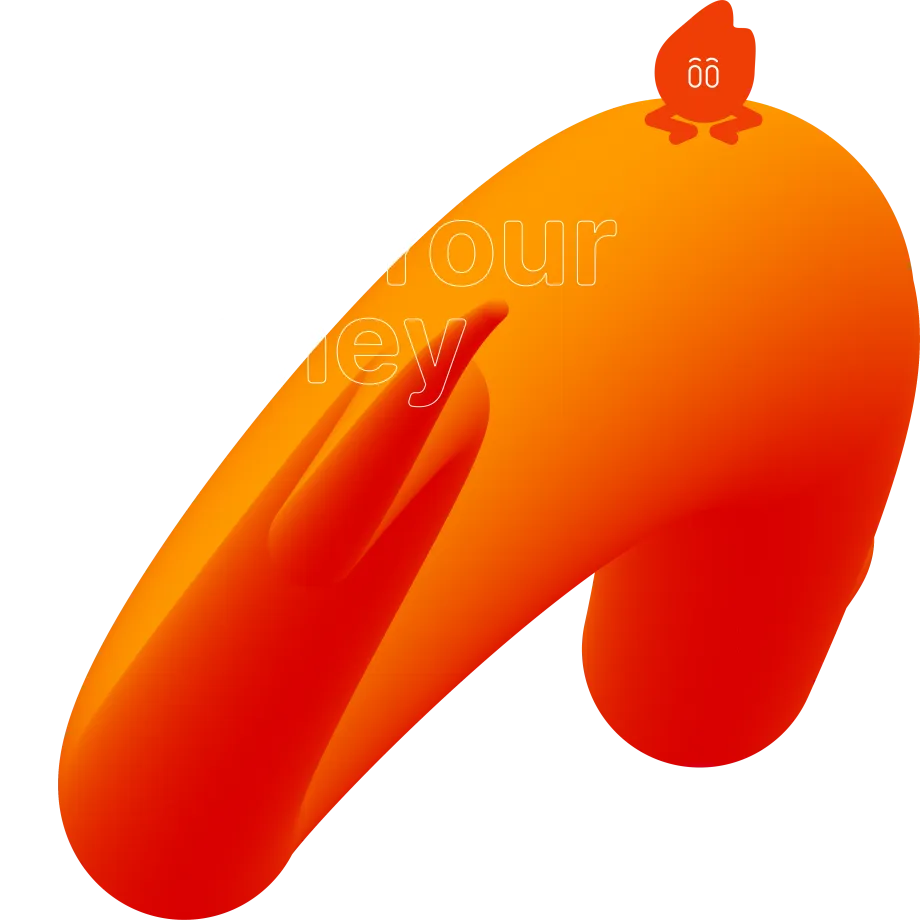
Begin Your Journey
01
Install Rust
The first step is to install Rust. Refer to the Rust book's installation page.
Create a Rust Application
- Open your preferred terminal or command prompt.
- Use Cargo, Rust's package manager, to create a new Burn application in a specific directory.
- Change your current directory to the newly created project
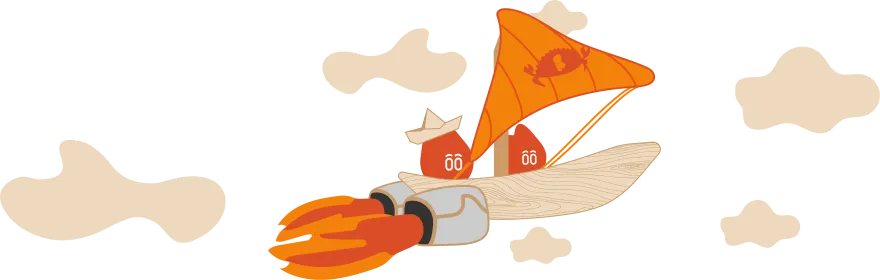
Add Burn Dependency and Choose Backend
- Cargo simplifies the process of including external libraries. The following command adds the main dependency and specify the [wgpu] backend
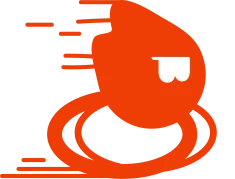
Start Developing with Burn
- With Burn successfully installed, you're now ready to start developing your deep learning projects using this powerful framework.
Basic Example
Now open [src/main.rs] and replace its content with:
use burn::tensor::{Tensor, backend::Backend};
fn computation<B: Backend>() {
// Create the device where to do the computation
let device = Default::default();
let tensor1: Tensor<B, 2> = Tensor::from_floats([[2., 3.], [4., 5.]], &device);
let tensor2 = Tensor::ones_like(&tensor1);
// Print the element-wise addition of the two tensors.
println!("{:}", tensor1 + tensor2);
}
fn main() {
computation::<burn::backend::Wgpu>();
}
And then:
You should now see the result of the addition:
Tensor {
data: [[3.0, 4.0], [5.0, 6.0]],
shape: [2, 2],
device: BestAvailable,
backend: "fusion<jit<wgpu<wgsl>>>",
kind: "Float",
dtype: "f32",
}
While this example is somewhat trivial, the basic workflow section of the Burn Book will walk you through a much more relevant example for deep learning applications.